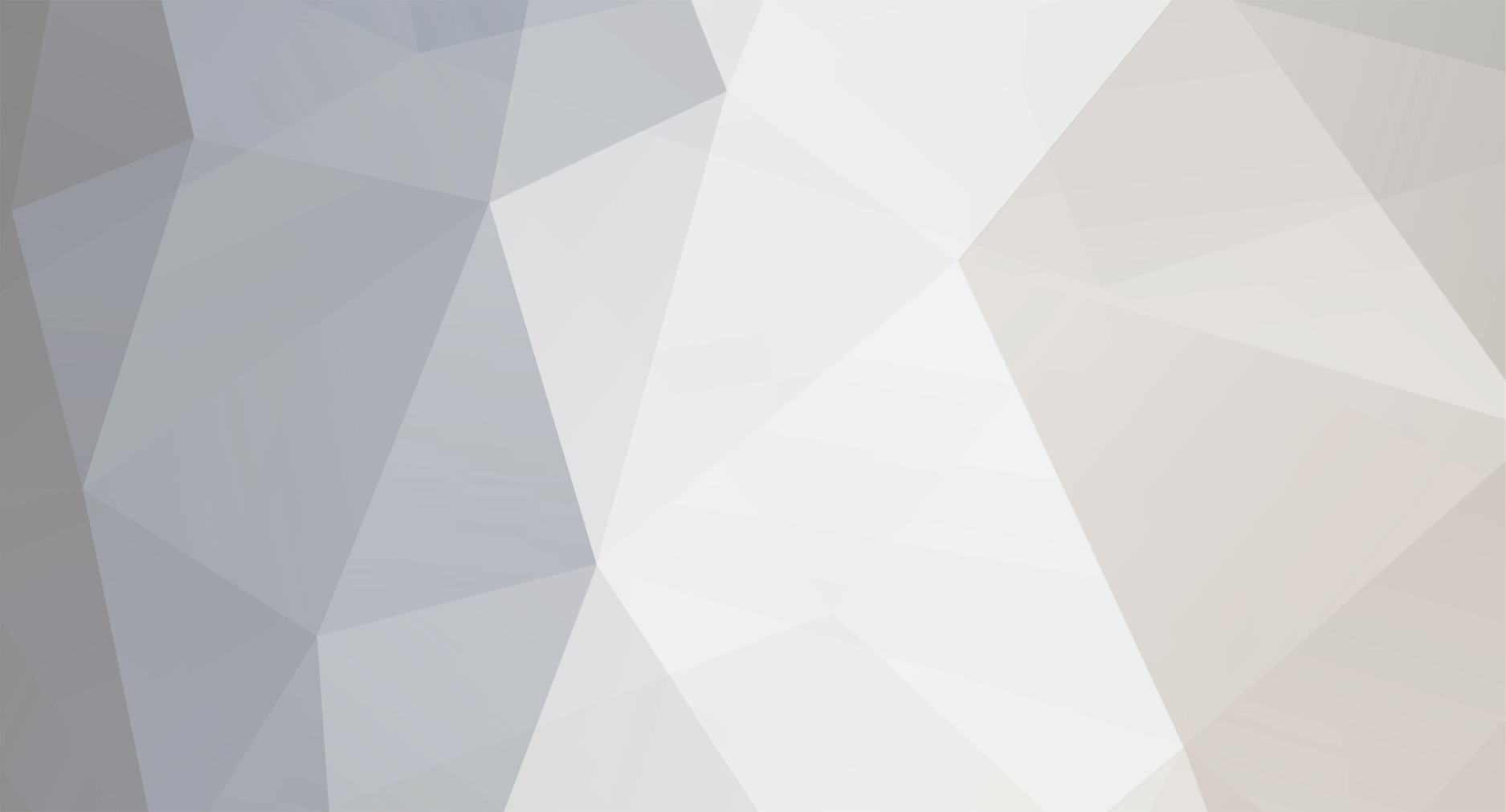
frostys11
Plebs-
Posts
5 -
Joined
-
Last visited
Everything posted by frostys11
-
Hey again, I'm implementing the script for the Aman'thuls Vision trinket and the procs are working correct although it procs from pretty much anything like mounting etc. Is there anyway I can change that? I know this might have to do with the spellfamily masks in the spellclassoptions.db2 file. However, how does bit mask matching actually work? I tried to add a new bit mask in the spell_proc table in trinitycore but was unable to get it to work correctly. I could hardcode it in the script files to trigger from the spells I want but that would be an ugly solution in my opinion. The second question is that I get the wrong stat from Aman'Thul's Grandeur buff (http://www.wowhead.com/spell=256832/amanthuls-grandeur). I noticed it has four different auraeffects. How can i cast a spell in trintycore with a specific aura effect to get the right stat? The third question is that I have implemented the Acrid Catalyst trinket proc and the logic works correctly but I'm getting 0 stat for the buffs and the proc chance seems to be slightly off. How could I fix these two things? I guess its a db thing since the stat needs to scale accroding to the ilvl version of the trinket? I tried to look a trinitycore documentation regarding this and I cant seem to find nor can I find it in the mangos documentation This is the code for the two trinket implementations: enum AcridCatalystInjector { SPELL_FERVOR_OF_THE_LEGION = 253261, // Haste SPELL_BURTALITY_OF_THE_LEGION = 255742, // Critical SPELL_MALICE_OF_THE_LEGION = 255744, // Mastery SPELL_CYCLE_OF_THE_LEGION = 253260 // Haste, Mastery, and Critical }; // Item - 151955: Acrid Catalyst Injector // 253259 - Item - Antorus, the Burning Throne (End raid of legion expansion) template <uint32 CriticalSpellId, uint32 HasteSpellId, uint32 MasterySpellId> class SpellItemAcridCatalystInjector : public SpellScriptLoader { public: SpellItemAcridCatalystInjector(char const* ScriptName) : SpellScriptLoader(ScriptName) { } template <uint32 Critical, uint32 Haste, uint32 Mastery> class SpellItemAcridCatalystInjectorAuraScript : public AuraScript { PrepareAuraScript(SpellItemAcridCatalystInjectorAuraScript); bool Validate(SpellInfo const* /*spellInfo*/) override { return ValidateSpellInfo( { Critical, Haste, Mastery }); } void HandleProc(AuraEffect const* aurEff, ProcEventInfo& eventInfo) { /*if (eventInfo.GetHitMask() & PROC_HIT_CRITICAL) {*/ static std::vector<uint32> const triggeredSpells[1] = { { Critical, Haste, Mastery } }; PreventDefaultAction(); Unit* caster = eventInfo.GetActor(); std::vector<uint32> const& randomSpells = triggeredSpells[0]; if (randomSpells.empty()) return; uint32 spellId = Trinity::Containers::SelectRandomContainerElement(randomSpells); caster->CastSpell(caster, spellId, true, nullptr, aurEff); for (std::vector<uint32>::const_iterator it = randomSpells.begin(); it != randomSpells.end(); ++it) { if (caster->HasAura((*it))) { auto trinketAura = caster->GetAura((*it)); if (trinketAura->GetStackAmount() >= 5) { caster->CastSpell(caster, SPELL_CYCLE_OF_THE_LEGION, true, nullptr, aurEff); caster->RemoveAura(Critical); caster->RemoveAura(Haste); caster->RemoveAura(Mastery); break; } } } //} } void Register() override { OnEffectProc += AuraEffectProcFn(SpellItemAcridCatalystInjectorAuraScript::HandleProc, EFFECT_0, SPELL_AURA_DUMMY); } }; AuraScript* GetAuraScript() const override { return new SpellItemAcridCatalystInjectorAuraScript<CriticalSpellId, HasteSpellId, MasterySpellId>(); } }; enum AmanThulsVision { SPELL_GLIMPSE_OF_ENLIGHTENMENT = 256818, SPELL_AMANTHULS_GRANDEUR = 256832 }; // Item - 154172: Aman'Thul's Vision // 256817 - Item - Antorus, the Burning Throne (End raid of legion expansion) template <uint32 GlimpseOfEnlightenmentSpellId, uint32 AmanthulsGrandeurSpellId> class SpellItemAmanThulsVision : public SpellScriptLoader { public: SpellItemAmanThulsVision(char const* ScriptName) : SpellScriptLoader(ScriptName) { } template <uint32 GlimpseOfEnlightenment, uint32 AmanthulsGrandeur> class SpellItemAmanThulsVisionAuraScript : public AuraScript { PrepareAuraScript(SpellItemAmanThulsVisionAuraScript); bool Validate(SpellInfo const* /*spellInfo*/) override { return ValidateSpellInfo( { GlimpseOfEnlightenment, AmanthulsGrandeur }); } void HandleProc(AuraEffect const* aurEff, ProcEventInfo& eventInfo) { static std::vector<uint32> const triggeredSpells[1] = { { GlimpseOfEnlightenment, AmanthulsGrandeur } }; PreventDefaultAction(); Unit* caster = eventInfo.GetActor(); caster->CastSpell(caster, GlimpseOfEnlightenment, true, nullptr, aurEff); //AuraEffect* test = caster->GetAuraEffect(256817, EFFECT_2, GetCasterGUID()); caster->CastSpell(caster, AmanthulsGrandeur, true, nullptr, aurEff); //<--- How do I get the specific aureffect from SPELL_AMANTHULS_GRANDEUR? //AuraEffect* test2 = caster->GetAuraEffect(SPELL_AMANTHULS_GRANDEUR, EFFECT_2, GetCasterGUID()); //caster->CastSpell(caster, AmanthulsGrandeur, true, nullptr, aurEff); } void Register() override { OnEffectProc += AuraEffectProcFn(SpellItemAmanThulsVisionAuraScript::HandleProc, EFFECT_0, SPELL_AURA_PROC_TRIGGER_SPELL); } }; AuraScript* GetAuraScript() const override { return new SpellItemAmanThulsVisionAuraScript<GlimpseOfEnlightenmentSpellId, AmanthulsGrandeurSpellId>(); } };
-
[Delete please] How to find area trigger ids
frostys11 replied to frostys11's topic in Help and Support
Nvm I figured it out -
Hey I'm new to trinitycore (not new to programming, I'm a java developer) and I'm trying to fix the volcanic inferno trait for elemental shaman in the ashamanecore repo and I'm trying to look on how other spells are implemented such as cinderstorm etc which its script name is binded in the areatrigger_template table. They all have an area trigger id which i cant seem to find in the db2 files I have generated. I have looked in the areatrigger table and all over the place in the db2 files and can't find anything related to area trigger ids for other spells commented in the code. Is this data that is sniffed from the client or? Also I'm a bit confused for example there is another talent for ench shaman called crashing storm which is a similiar area affect to the volcano which is triggered when you use crash lightning. However this is located in the spell_areatrigger and the script name is in areatrigger_scripts. I'm trying to wrap my head around how all the fields and all the tables go together with the code and what all the flags and enum values mean. How does it know to trigger the spell with a specific id from the area trigger id?
-
Yeah thanks so much forgot to mention that I solved it. Yeah just built the install folder and it built out to the folder I specified with the prefix. It works great for the ashmancore btw!
-
Hey I'm trying to configure hot reloading that Naios (Denis Blank) implemented a while ago to speed up development. The step I am confused at which is probably really easy and really trivial is when he says to make sure to change the target to the install target in visual studio to install to the CMAKE_INSTALLPREFIX location. Can anyone clarify this? Screenshot provided below and highlighted with red border its step 2 I'm confused with